s0257
This commit is contained in:
parent
495a134494
commit
0efe4e243c
17
include/s0257_binary_tree_paths.hpp
Normal file
17
include/s0257_binary_tree_paths.hpp
Normal file
@ -0,0 +1,17 @@
|
||||
#ifndef S0257_BINARY_TREE_PATHS_HPP
|
||||
#define S0257_BINARY_TREE_PATHS_HPP
|
||||
|
||||
#include <queue>
|
||||
#include <string>
|
||||
#include <vector>
|
||||
|
||||
#include "structures.hpp"
|
||||
|
||||
using namespace std;
|
||||
|
||||
class S0257 {
|
||||
public:
|
||||
vector<string> binaryTreePaths(TreeNode* root);
|
||||
};
|
||||
|
||||
#endif
|
@ -2,7 +2,7 @@
|
||||
|
||||
## 二叉树的种类
|
||||
|
||||
满二叉树:如果一棵二叉树只有度为0的结点和度为2的结点,并且度为0的结点在同一层上,则这棵二叉树为满二叉树。
|
||||
满二叉树:如果一棵二叉树只有度为 0 的结点和度为 2 的结点,并且度为 0 的结点在同一层上,则这棵二叉树为满二叉树。
|
||||
|
||||
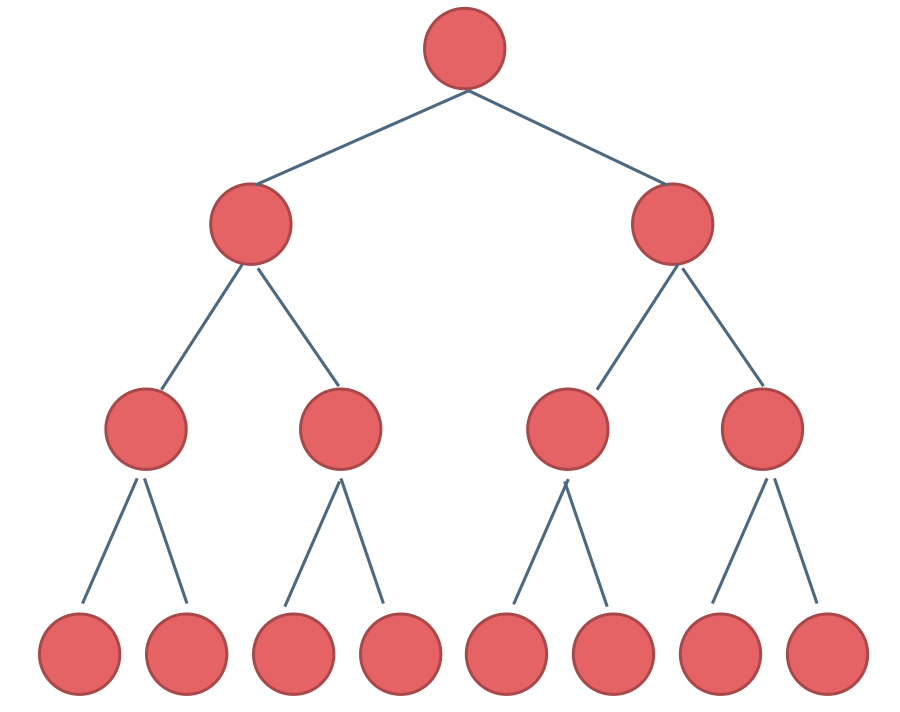
|
||||
|
||||
@ -18,7 +18,7 @@
|
||||
|
||||
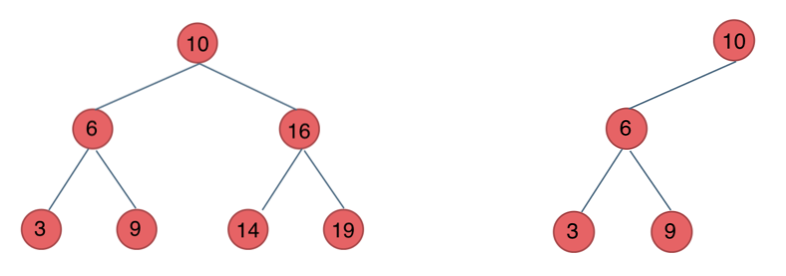
|
||||
|
||||
平衡二叉搜索树:又被称为AVL(Adelson-Velsky and Landis)树,且具有以下性质:它是一棵空树或它的左右两个子树的高度差的绝对值不超过1,并且左右两个子树都是一棵平衡二叉树。
|
||||
平衡二叉搜索树:又被称为 AVL(Adelson-Velsky and Landis)树,且具有以下性质:它是一棵空树或它的左右两个子树的高度差的绝对值不超过 1,并且左右两个子树都是一棵平衡二叉树。
|
||||
|
||||
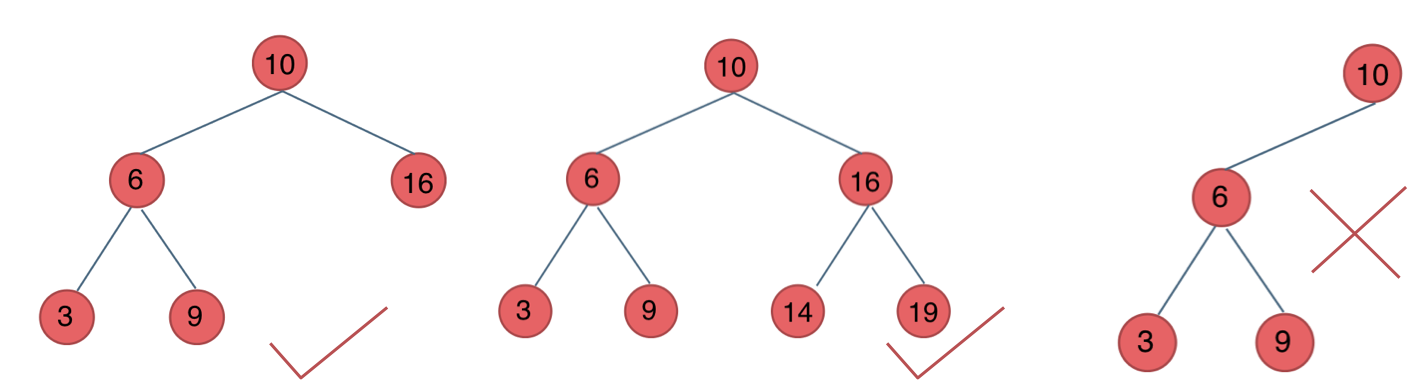
|
||||
|
||||
@ -39,7 +39,7 @@ struct TreeNode {
|
||||
|
||||
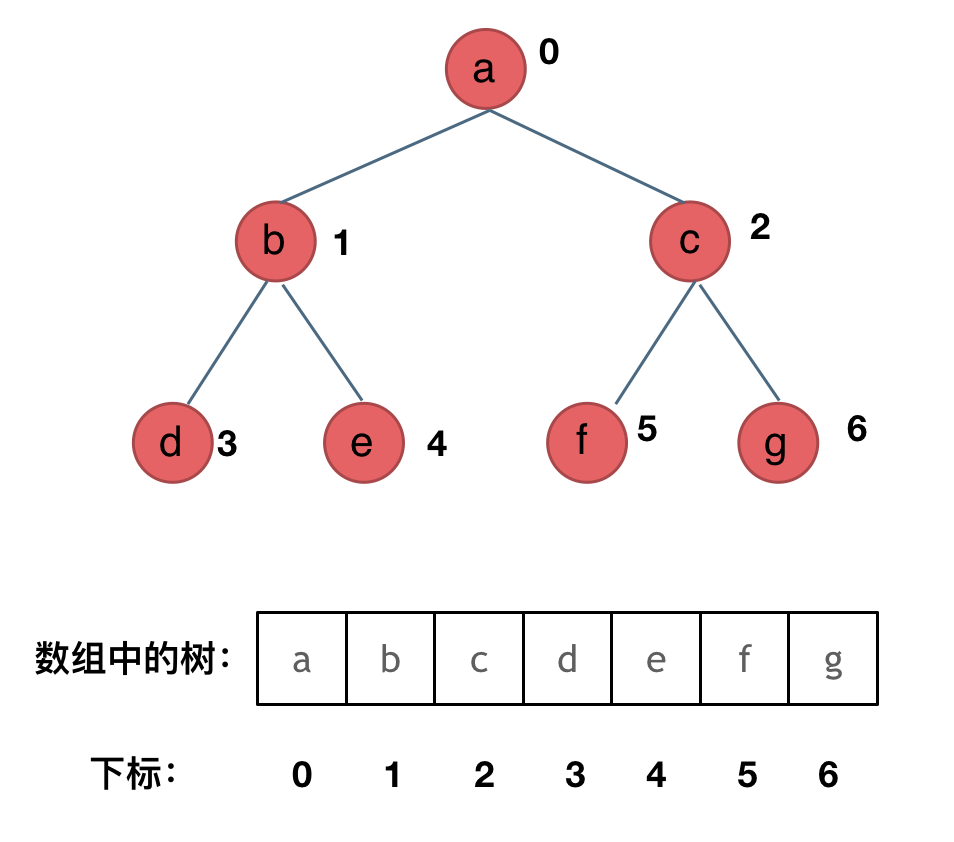
|
||||
|
||||
如果父节点的数组下标是 i,那么它的左孩子就是 i * 2 + 1,右孩子就是 i * 2 + 2。
|
||||
如果父节点的数组下标是 `i`,那么它的左孩子就是 `i * 2 + 1`,右孩子就是 `i * 2 + 2`。
|
||||
|
||||
## 遍历方式
|
||||
|
||||
@ -67,3 +67,7 @@ struct TreeNode {
|
||||
- 前序遍历:中左右
|
||||
- 中序遍历:左中右
|
||||
- 后序遍历:左右中
|
||||
|
||||
## 技巧
|
||||
|
||||
1. 深度优先搜索从下往上,广度优先搜索从上往下,所以如果需要处理从上往下并且状态积累的情形 (e.g. [s0404](https://leetcode.cn/problems/sum-of-left-leaves/) && [s0257](https://leetcode.cn/problems/binary-tree-paths/)) 可以先创建一个结构体用来描述节点状态,然后用 BFS 遍历。
|
||||
|
27
src/s0257_binary_tree_paths.cpp
Normal file
27
src/s0257_binary_tree_paths.cpp
Normal file
@ -0,0 +1,27 @@
|
||||
#include "s0257_binary_tree_paths.hpp"
|
||||
|
||||
struct TreeNodeState_S0257 {
|
||||
TreeNode* ptr;
|
||||
string path;
|
||||
};
|
||||
|
||||
vector<string> S0257::binaryTreePaths(TreeNode* root) {
|
||||
queue<TreeNodeState_S0257> q;
|
||||
vector<string> result{};
|
||||
if (root) q.push(TreeNodeState_S0257{root, std::to_string(root->val)});
|
||||
while (!q.empty()) {
|
||||
TreeNodeState_S0257 front = q.front();
|
||||
q.pop();
|
||||
if (front.ptr->left == nullptr && front.ptr->right == nullptr)
|
||||
result.push_back(front.path);
|
||||
if (front.ptr->left)
|
||||
q.push(TreeNodeState_S0257{
|
||||
front.ptr->left,
|
||||
front.path + "->" + std::to_string(front.ptr->left->val)});
|
||||
if (front.ptr->right)
|
||||
q.push(TreeNodeState_S0257{
|
||||
front.ptr->right,
|
||||
front.path + "->" + std::to_string(front.ptr->right->val)});
|
||||
}
|
||||
return result;
|
||||
}
|
Loading…
x
Reference in New Issue
Block a user